PROJECT: Cow
Overview
Cow is a desktop project management application where you can manage both tasks and contacts, as well as assign tasks to contacts and vice versa. It also features a calendar to provide an easy visual reference of tasks chronologically.
Cow has an emphasis on interaction using Command Line Interface ("CLI"). It is written in Java, and it has a Graphical User Interface ("GUI") created with JavaFX. Cow consists of approximately 14k LoC in Java.
Cow used Address Book (Level 4) as a base for development. |
Summary of contributions
-
Major enhancement: added the ability to edit, delete and batch delete tasks
-
What it does: This feature allows you to edit tasks as well as delete one or more tasks at a time
-
Justification: This feature improves the product significantly because sometimes you may add duplicate tasks or add tasks with wrong details, thus task editing is essential. Moreover, sometimes you may need to delete more than one tasks at a time. Instead of doing it one-by-one which is time-consuming, they can do it in one command instead.
-
Highlights: This enhancement was built such that the command format between tasks and contacts are similar to improve your experience. Moreover, the batch delete tasks syntax is also built on top of the original single delete command. Efforts were taken to ensure that code duplication remains minimum between delete and batch delete to ensure that the code is maintainable.
-
-
Minor enhancement 1: Updated sample tasks to include tasks as well assignment between some tasks and contacts
-
What it does: Upon initial launch, these sets of contacts, tasks and assignment thereof are loaded as default data.
-
Justification: To get familiar with Cow, it is good to have some sample data to play and experiment with. Once you are ready, you can run
clear
to clear all the data in Cow. -
Highlights: This enhancement required me to write a helper function inside
SampleDataUtil
to do assignments between tasks and contacts. This is to ensure data integrity by doing a two-way assignment (task-to-person and person-to-task) as one transaction.
-
-
Minor enhancement 2: Added tasks select command to select a task and show its details in the task details pane.
-
What it does: It selects a task from the displayed task list, and show the task in the task details pane.
-
Justification: The space available in the tasks lists is very small, so most times the task name is cut off. In order to see more details, you need a command to select from that list to show in the task details pane.
-
Highlights: This enhancement required me to use an Event already wrote by my teammate,
JumpToTaskListRequestEvent
. In order to use it, I need to be able to understand the documentation written by my teammate. This showcases my ability to work in with modular code written by several developers.
-
-
Code contributed: Collated code at RepoSense
-
Other contributions:
-
Project Management:
-
Managed releases
v1.3.2
andv1.4
(2 releases) on GitHub -
Managed milestone
v1.4
issue tracker on GitHub
-
-
Enhancements to existing features:
-
Enlarged headless framebuffer for GUI tests to 1920x1080 so that GUI unit tests will not fail in Travis CI: #183
-
Refactor all branding from AddressBook to Cow: #183
-
Made sure that when the main window opens, it is big enough to show the calendar pane: #183
-
Added module name in usage messages: #87
-
Allow editing task’s either start or end DateTime: #94
-
-
Documentation:
-
Totally revamped the User Guide: #158
-
Updated the User Guide to include new features: #84
-
Updated the UI class diagram in the Developer Guide: #172
-
Updated the Developer Guide to include the use case of the new features implemented: #84
-
Added diagram to explain how the new features included were implemented: #62
-
-
Community:
-
Tools:
-
Integrated a new GitHub plugin (coveralls) to the project to track test coverage (#50)
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Quick Start
-
Ensure you have JRE 9 or later installed.
-
Download the latest
.jar
file here. -
Place it in the folder where you want the data files to be stored.
-
Double-click the
.jar
file to start the app. The GUI should appear in a few seconds -
Type in a command and press Enter to execute it. For a start, type
help
and press Enter to see the help page. -
Some example commands you can try:
-
contacts list
: lists all contacts -
contacts add n/John Doe p/98765432 e/johnd@example.com a/311, Clementi Ave 2, #02-25 t/friends t/owesMoney
: adds contact namedJohn Doe
to Cow -
tasks list
: lists all tasks -
tasks delete 2
: deletes the 2nd task shown in the list -
exit
: exits Cow
-
Ensure that your screen resolution is at least 1920x1080, or the calendar might not show properly. |
Edit task: tasks edit
Edits an existing task in Cow.
Format:
tasks edit INDEX [n/TASK_NAME] [sd/START_DATE st/START_TIME] [ed/END_DATE et/END_TIME] [t/TAG]…
Examples:
-
tasks edit 1 sd/20050108 st/1235
Edits the start date and time of the 1st task to be 8 January 2005, 12:35pm. -
task edit 2 n/Brush the cows t/
Edits the name of the 2nd task to beBrush the cows
and clears all existing tags.
Delete task: tasks delete
Deletes all tasks corresponding to the indices provided.
Format: tasks delete INDEX1 [INDEX2] [INDEX3] …
Examples:
-
tasks delete 1
Deletes the 1st task on the displayed task list -
tasks delete 1 5 4
Deletes the 1st, 4th and 5th tasks in the displayed task list
Delete all tasks: tasks delete all
Deletes all tasks that are shown in the displayed task list.
Format: tasks delete all
If you accidentally deleted all tasks in the displayed task list, use undo to
recover deleted tasks.
|
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
UI component
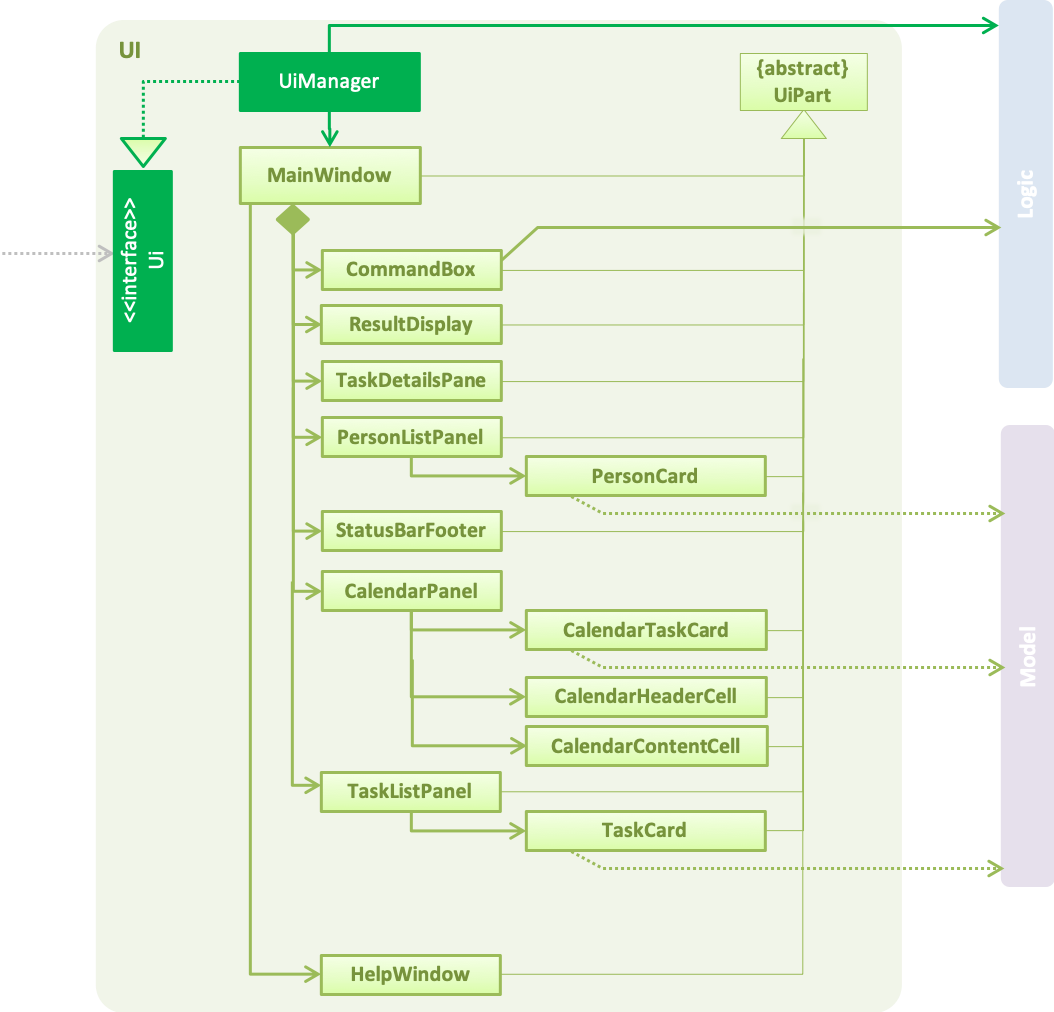
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, StatusBarFooter
, etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
Additionally, the CalendarPanel
consists of CalendarHeaderCell
to indicate days of week, CalendarContentCell
to indicate the date, and CalendarTaskCard
for each of the tasks that falls on that date
The UI
component,
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
so that the UI can auto-update when data in theModel
change. -
Responds to events raised from various parts of the App and updates the UI accordingly.
Editing and Batch Deleting Tasks
Current implementation
The code for editing and deleting tasks is actually pretty similar to how it is implemented for persons. This is a combination of adding support for two additional commands: tasks edit
and tasks delete
inside TasksParser
, defining the parser classes EditCommandParser
and DeleteCommandParser
, and the command classes EditCommand
and DeleteCommand
, and finally, adding void updateTask(Task target, Task editedTask);
and void deleteTask(Task target);
in the Model
interface, and implementing them in the ModelManager
class.
As for validation, in EditCommandParser
, user input will be checked whether they conform to the format, else ParseException
is thrown and usage message will be shown to the user. Meanwhile, EditCommand
does another kind of validation: that the start date and time are before the end date and time, else CommandException
is thrown and the user will be informed to ensure the chronological order of start and end DateTime
. All these validations are done using methods defined inside DateTime
.
To explain more clearly, you can see below a sequence diagram of what happens when the user asks the program to edit a task:
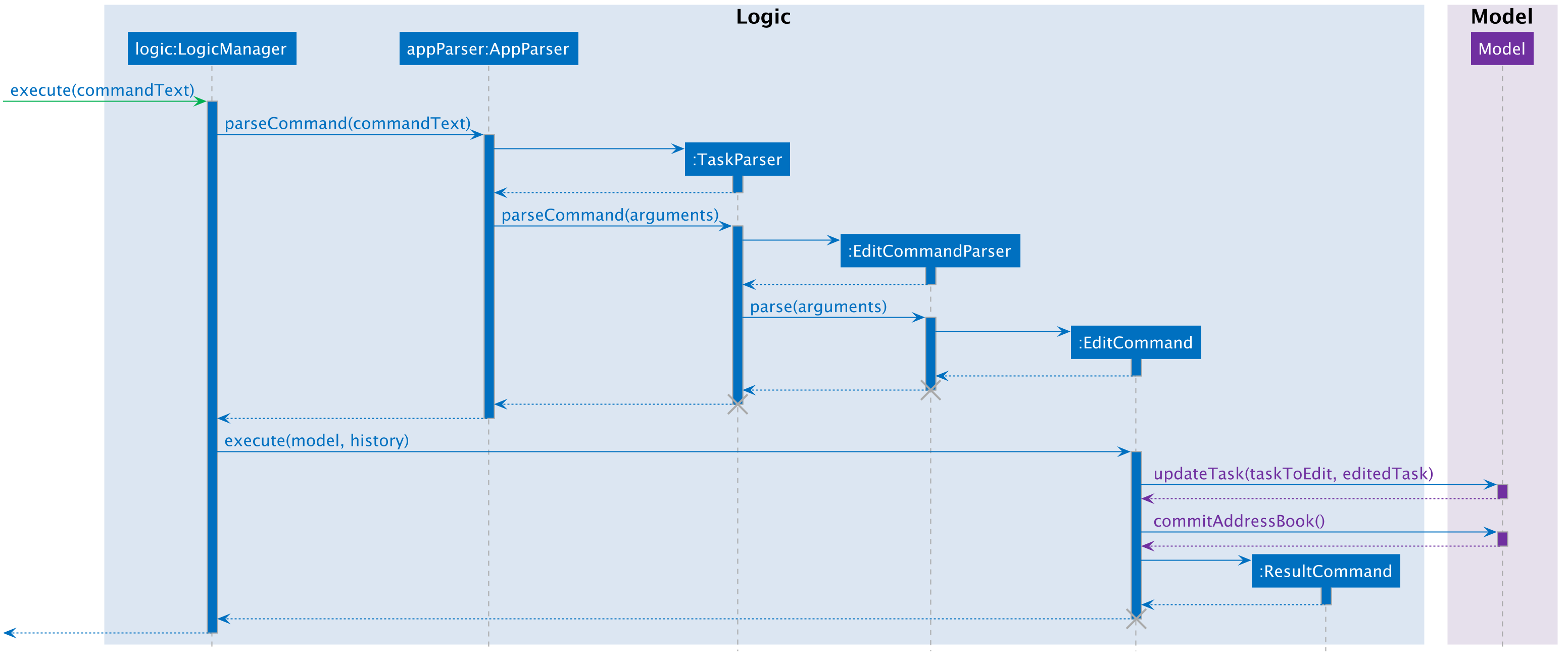
tasks edit
Command in the Logic
Component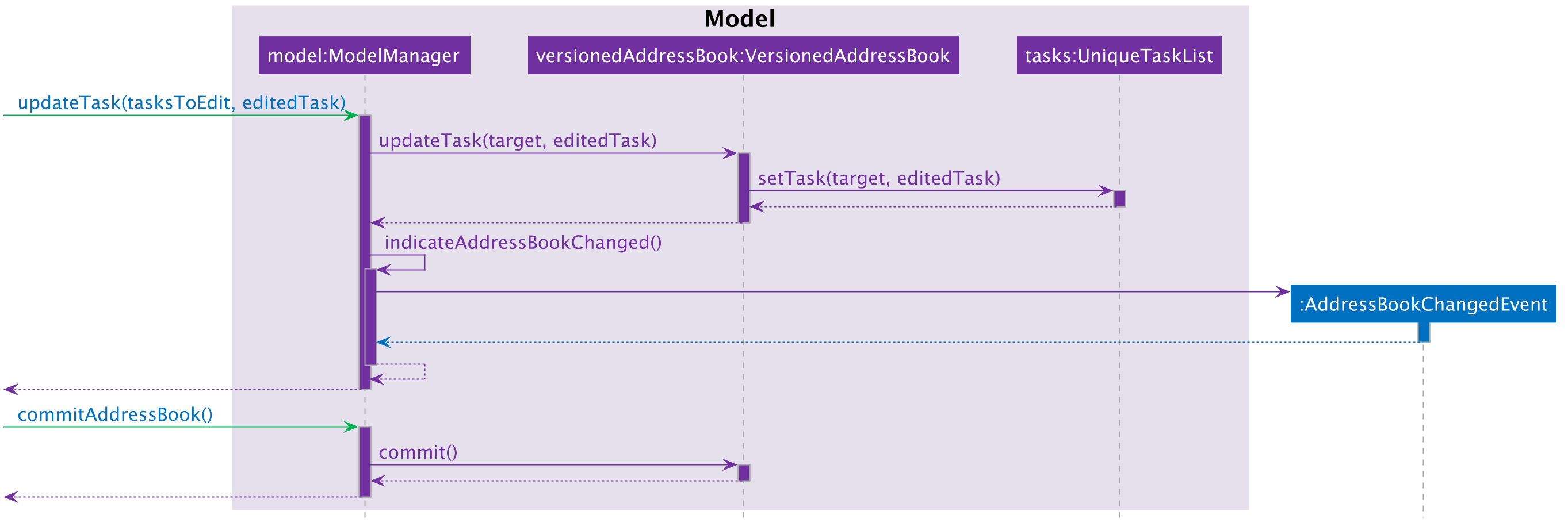
tasks edit
Command in the Model
ComponentPlease do note that DeleteCommand
for Task
is different from that of Person
in that the former actually takes in a list of indices to be deleted, which is required to support batch deleting tasks. Changes are also made in DeleteCommandParser
to add support for multiple indices user input, and to recognise the keyword "all"
. Should the keyword "all"
be encountered, the list passed to DeleteCommand
is null
, which is a special value to indicate to DeleteCommand
to delete all tasks in the current displayed list. You can find below the sequence diagram for batch-deleting task(s).
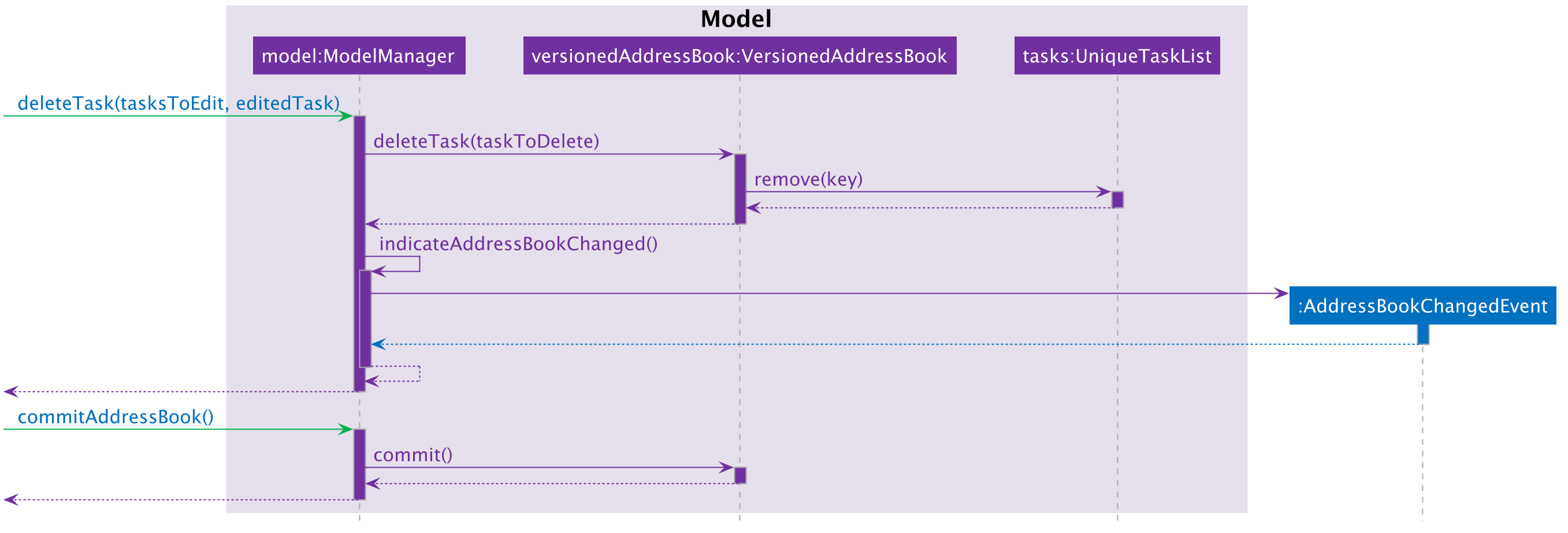
tasks delete
Command in the Logic
Component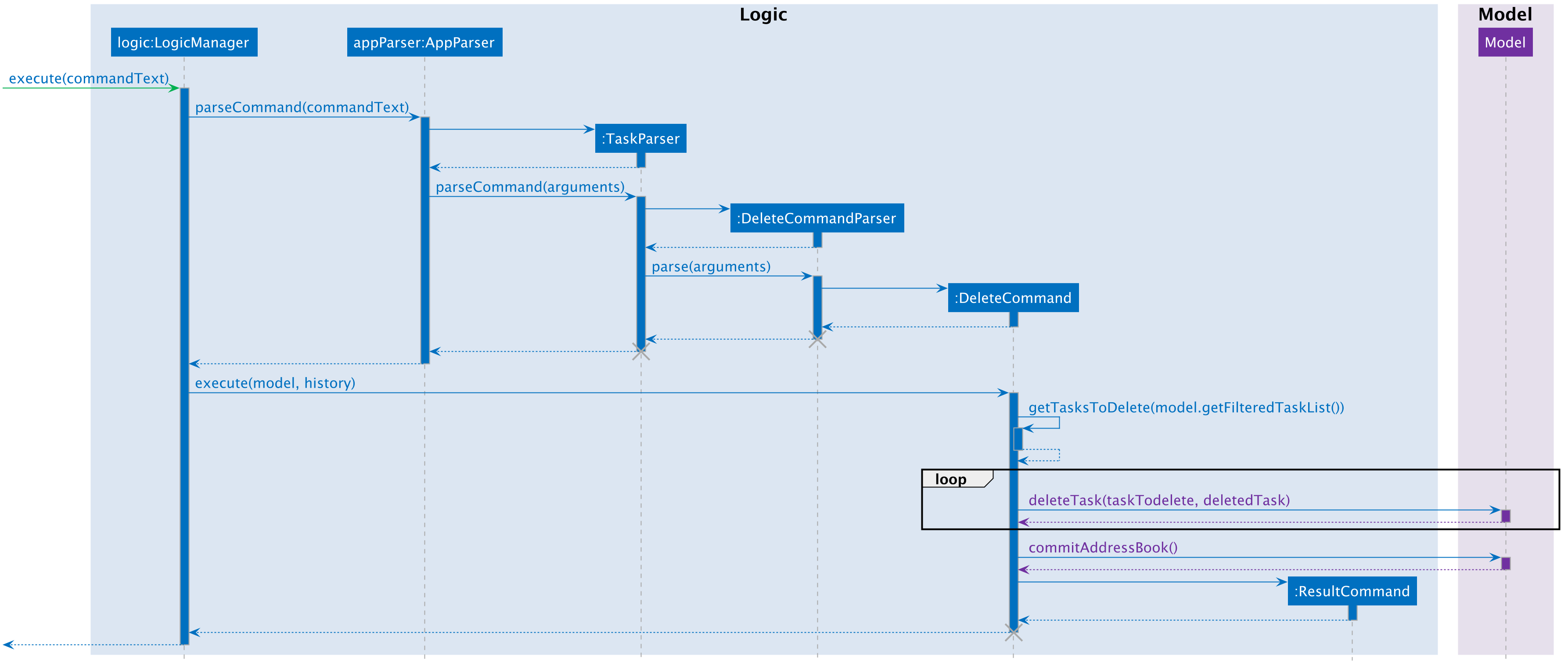
tasks delete
Command in the Model
Component
These diagrams are generated using PlantUML. The files used to generate the image files are stored inside docs/plantuml .
|
Note the AddressBookChangedEvent
that is raised — this will notify StorageManager
that there is a change in the AddressBook
, and so to save to disk.
Future Enhancements
Currently, the methods void updateTask(Task target, Task editedTask)
and void updatePerson(Person target, Person editedPerson)
in the Model
interface are very similar. Perhaps in the future Model
can be refactored as a generic so that there is minimum code duplication.
Edit a task
MSS
-
User requests to list tasks.
-
Cow shows a list of tasks.
-
User requests to edit a specific task in the list, together with the new attributes.
-
Cow edits the task with the given attribute.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. User provides an invalid index.
-
3a1. Cow shows an error message.
Use case resumes at step 2.
-
-
3a. User provides an invalid attribute.
-
3a1. Cow shows an error message.
Use case resumes at step 2
-
Delete a task
MSS
-
User requests to list tasks.
-
Cow shows a list of tasks.
-
User requests to delete a specific task in the list.
-
Cow deletes the task.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends
-
3a. User provides an invalid index.
-
3a1. Cow shows an error message.
Use case resumes at step 2
-
Batch delete tasks
MSS
-
User requests to list tasks.
-
Cow shows a list of tasks.
-
User requests to delete a number of tasks in the list.
-
Cow deletes the specified tasks.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. User provides a list of indices containing an invalid index.
-
3a1. Cow shows an error message. Use case resumes at step 2
-
Delete all tasks
MSS
-
User requests to list tasks.
-
Cow shows a list of tasks.
-
User requests to delete all tasks in the list.
-
Cow deletes all tasks in the list.
Appendix A: Instructions for Manual Testing
Given below are instructions to test the app manually.
Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample contacts and tasks. The window size should be optimum if your screen’s resolution is at least 1920x1080.
-
-
Saving window preferences
-
In case window size is not optimum, resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
-
Closing the application
-
Test case:
exit
Expected: the application should exit and the window should disappear.
-
Adding Tasks
-
Adding a task while all tasks are listed
-
Prerequisites: List all tasks using the
tasks list
command. There can be any number of tasks in the list. -
Test case:
tasks add n/Brush the cows sd/20180101 st/0000 ed/20181231 et/2359 t/Chill
Expected: A new task with the fields entered is added to the bottom of the task list. -
Test case:
tasks add n/Slaughter the cows ed/20190101 et/1200
Expected: A new task with the entered name and end date and time is added with the current date and time as the start date and time. -
Test case:
tasks add n/Feed the cows sd/20180101 st/2359 ed/20180101 et/0000
Expected: No task is added. An error message is shown. -
Test case:
tasks add n/Feed the cows ed/19700101 et/0000
Expected: No task is added. An error message is shown. -
Test case:
tasks add n/Feed the cows sd/20180101 st/0000 ed/20180231 et/2500
Expected: No task is added. An error message is shown. -
Other incorrect tasks add commands to try: Names containing non-alphanumeric characters, dates that don’t exist on the calendar, times outside the range 0000-2359 inclusive, wrongly formatted dates and times.
-
Editing Tasks
-
Editing a task while all tasks are listed
-
Prerequisites: List all tasks using the
tasks list
command. There are at least 4 tasks in the list. -
Test case:
tasks edit 1 n/Slaughter the cow
Expected: First task is edited such that its name is nowSlaughter the cow
. New details of the edited task are shown in the status message. Timestamp in the status bar is updated. -
Test case:
tasks edit 1 sd/20180101 st/0000 ed/20180101 et/2359
Expected: First task is edited such that its start date and time are 1 January 2018, 00.00am and its end date and time are 1 January 2018, 23.59pm. New details of the edited task are shown in the status message. Timestamp in the status bar is updated. -
Test case:
tasks edit 1 t/
Expected: First task is cleared of any tags that it might have had. New details of the edited task are shown in the status message. Timestamp in the status bar is updated. -
Test case:
tasks edit 1 t/messy
Expected: First task’s tag is now onlymessy
. New details of the edited task are shown in the status message. Timestamp in the status bar is updated. -
Test case:
tasks edit 1 t/messy t/mean
Expected: First task’s tags are nowmean
andmessy
. They are shown in no particular order. New details of the edited task are shown in the status message. Timestamp in the status bar is updated. -
Other correct tasks edit command to try: Combining editing name with start and/or end date and time and/or tags, Editing only start date and time as long as they are before the end date and time.
-
Test case:
tasks edit 0 n/Slaughter the cow
Expected: No task is edited. Error detail is shown in the status message. Status bar remains the same. -
Test case:
tasks edit 1 sd/20181231 st/0000 ed/20180101 et/2359
Expected: No task is edited. Error detail is shown in the status message. Status bar remains the same. -
Test case:
tasks edit 1
Expected: No task is edited. Error detail is shown in the status message. Status bar remains the same. -
Other incorrect tasks edit command to try:
tasks edit
,tasks edit x
(where x is negative or larger than the list size), Editing with invalid start and/or date and/or time, Editing with non-alphanumeric characters in the task name.
-
Deleting Task(s)
-
Deleting a task while all tasks are listed
-
Prerequisites: List all tasks using the
tasks list
command. There are at least 4 tasks in the list. -
Test case:
tasks delete 1
Expected: First task is deleted from the task list. Details of the deleted task are shown in the status message. Timestamp in the status bar is updated. -
Test case:
tasks delete 0
Expected: No task is deleted. Error detail is shown in the status message. Status bar remains the same. -
Other incorrect tasks delete command to try:
tasks delete
,tasks delete x
(where x is negative or larger than the list size)
-
-
Deleting multiple tasks while all tasks are listed
-
Prerequisites: List all tasks using the
tasks list
command. There are at least 4 tasks in the list. -
Test case:
tasks delete 1 3 2
Expected: First, second and third tasks are deleted from the task list. Details of the deleted tasks are shown in the status message. Timestamp in the status bar is updated. -
Test case:
tasks delete 1 4 0
Expected: No task is deleted. Error detail is shown in the status message. Status bar remains the same. -
Other incorrect tasks delete command to try:
tasks delete 1 2 x
(where x is negative or larger than the list size)
-
-
Deleting all tasks shown in the task list
-
Prerequisites: List all tasks using the
tasks list
command. There are at least 1 task. -
Test case:
tasks delete all
Expected: All tasks shown in the task list are deleted. Details of the deleted tasks are shown in the status message. Timestamp in the status bar is updated.
-