Overview
Cow is a task management program with a view towards team projects. It includes an integrated contacts management module and assignment of tasks to contacts. Its command-line interface (CLI) is geared towards power users, letting them carry out operations efficiently without their hands leaving the keyboard.
Cow was developed by a team of five Computer Science students from the National University of Singapore, including myself. This project portfolio documents my personal contributions to this project, including 1.5k lines of code (LoC) and contributions to user and developer documentation.
Summary of contributions
This section documents the features I contributed to the project.
-
Major enhancement: added structural scaffolding for the tasks module
-
Created the logic for tasks. This included creating a parser for task commands, and implementing a two-level parser to direct user commands the parser for either the contacts module or the tasks module, maintaining modularity of the parsers.
-
Created the model for tasks. This included auxillary classes including a DateTime class containing input validation and formatted text output tailored to this project.
-
-
Major enhancement: added ability to create tasks in Cow
-
Created a command for creating a task.
-
Made entry of start date and time of the task optional, defaulting to the date and time of the current moment. This enables faster entry of tasks that are already in progress.
-
-
Code contributed: https://nus-cs2103-ay1819s1.github.io/cs2103-dashboard/#=undefined&search=zxjtan
Contributions to the User Guide
This section includes my contributions to the user guide, displaying my ability to write user-targeted documentation. |
Add task: tasks add
Adds a task to Cow.
Format:
tasks add n/TASK_NAME [sd/START_DATE] [st/START_TIME] ed/END_DATE et/END_TIME [t/TAG]…
If start date or time is not entered, the missing field(s) will default to the current date/time. Therefore, it is invalid to enter an end date and time before the current date and time. |
Examples:
-
tasks add n/Math Assignment sd/20180101 st/0000 ed/20181231 et/2359 t/school t/urgent
-
tasks add n/Milk the cows ed/20181129 et/2359 t/farm
Contributions to the Developer Guide
You may see below my contributions to the developer guide, showcasing my technical writing skills as well as the technical depth of my code contributions. |
Design
Logic component
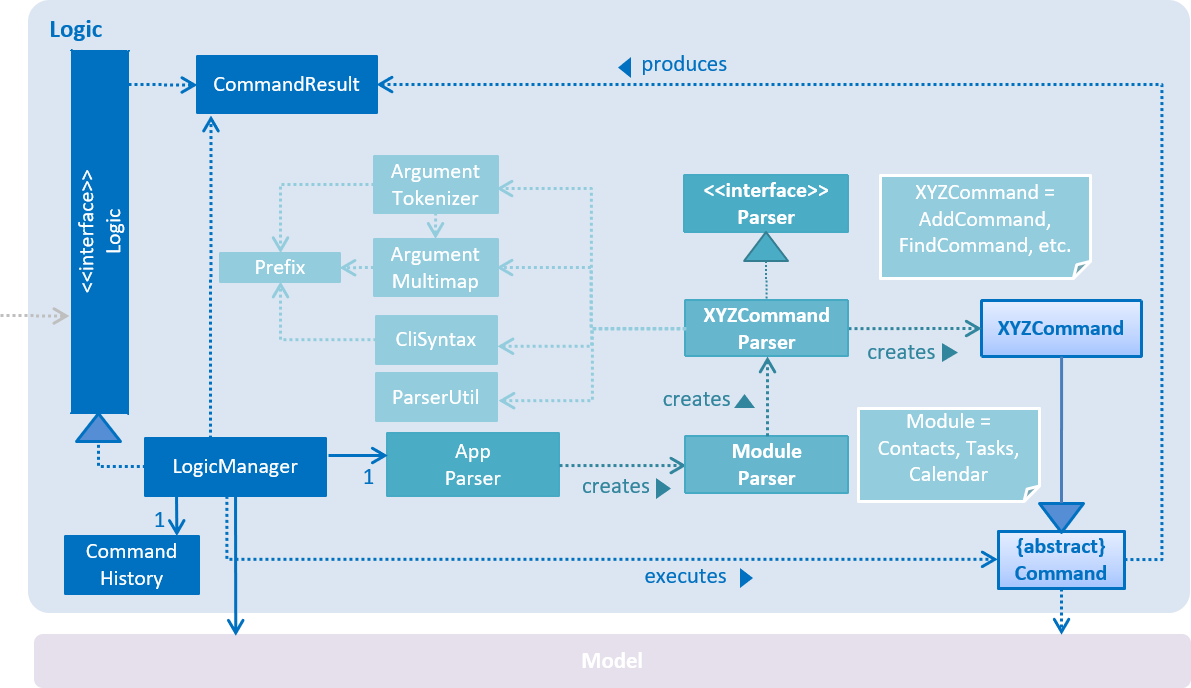
API :
Logic.java
-
Logic
uses theAppParser
class to parse the user command. -
AppParser
then sends the command to the appropriate module parser. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a person) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("contacts delete 1")
API call.
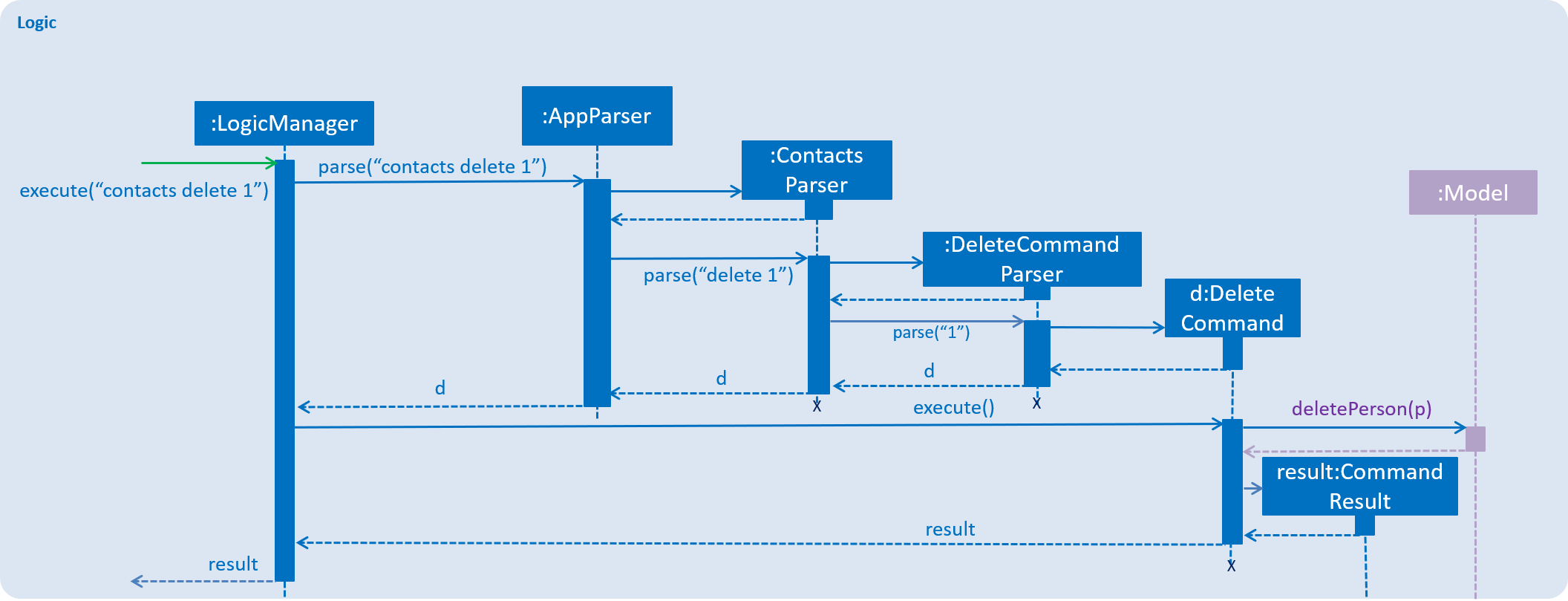
contacts delete 1
CommandModel component
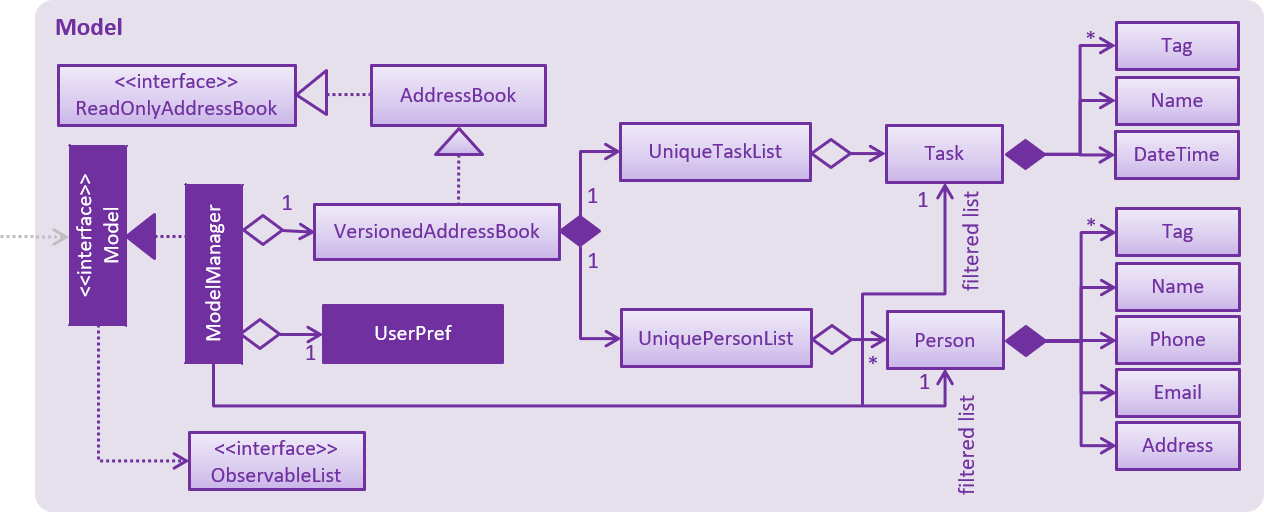
API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores Person and Task data.
-
exposes an unmodifiable
ObservableList<Person>
andObservableList<Task>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
Implementation
Task class
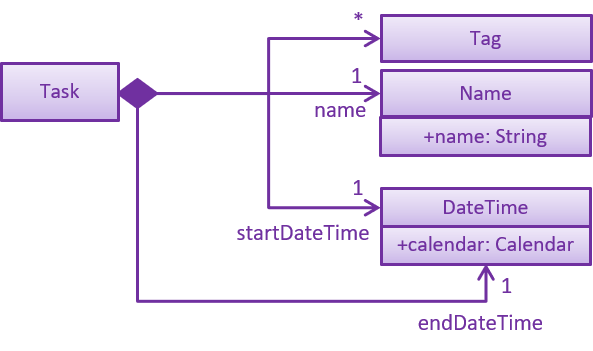
The Task
class consists of list of Tag
objects and a Name
object, corresponding to the same classes in the Person
namespace. It also has two DateTime
objects, one for the start date and time and the other for the end date and time of the task.
The DateTime
class encapsulates a Java Calendar
object to store a date and time. It also includes methods to parse and validate date/time inputs from the user, and methods to return the date or time as a String
.
Addition of tasks
Adding a task is fairly straightforward. The user command is given to the parser, which validates the input and creates the task and the objects it is associated with. One aspect with a more involved implementation is the accomodation of optional start date and time fields in the user input, which is illustrated here.
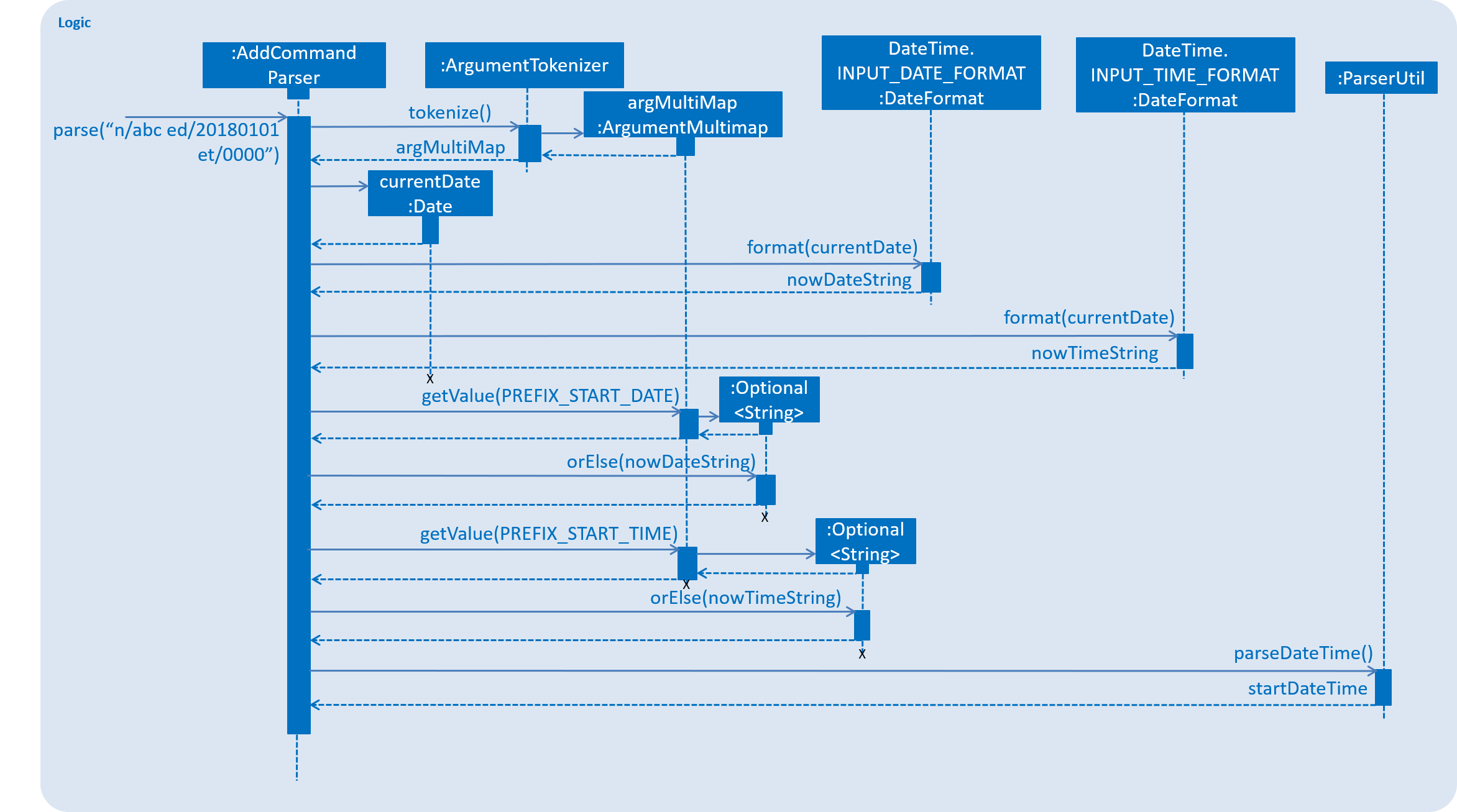
-
The
AddCommandParser
receives the input string and callsArgumentTokenizer.tokenize()
to create anArgumentMultiMap
. -
It then creates a Date object for the current moment in time and uses the
INPUT_DATE_FORMAT
andINPUT_TIME_FORMAT
DateFormat
s inDateTime
to parse the Date object into date and time strings in the correct format. -
It then retrieves the start date and start time strings from
argMultiMap
, which returns anOptional<String>
for each of them. -
orElse()
is then called on eachOptional<String>
to obtain the string encapsulated by theOptional
, or the string for the current date/time if theOptional
is empty. -
Finally,
ParserUtil.parseDateTime()
is called with the resultant date and time strings.
User Stories
While the whole group contributed to the table of user stories, I was the one who collated it at the end, streamlining redundancies and standardising the format and grammar. |
Priorities: High (must have) - * *
, Medium (nice to have) -
,
Low (unlikely to have) -
Priority | As a… | I want to… | So that I can… |
---|---|---|---|
|
user |
CRUD Tasks |
|
|
project manager |
assign other tasks to people |
track who’s supposed to complete them and notify them that they are supposed to complete the task |
|
project manager |
see the tasks assigned to each person |
know what they’re supposed to do |
|
project manager |
see an overview of all tasks and people assigned |
get a sense of the state of my project and tasks |
|
existing user |
search for people and tasks |
quickly find the person or task I watn |
|
existing user |
group tasks according to categories/tags |
manage a larger number of tasks easily |
|
existing user |
be alerted to tasks near their deadline |
complete tasks on time |
|
project manager |
send email notifications to people I assigned tasks to |
ensure they are on track with tasks |
|
user |
see milestones visualised using a calendar |
have a more chronological sense of my tasks |
|
user |
view team members’ calendars and share my own |
coordinate meetings and track progress |
|
user |
track the extent of others’ involvement in each task |
ensure fair distribution of credit |
|
user |
use this product as a web app |
easily access my tasks/work on all platforms |
|
user |
create recurring tasks |
avoid repeatedly creating a new task for each recurrence |
|
user |
have Autocomplete when typing tags |
find existing tags faster |
|
power user |
create command aliases |
enter commands more efficiently |
|
power user |
vim-mode CLI |
enter commands more efficiently |
|
power user |
emacs-mode CLI |
enter commands more efficiently |
Use cases
Create a task
MSS
-
User requests to add a task, together with all the attributes.
-
Cow adds that task.
Use case ends.
Extensions
-
1a. User provides an invalid attribute.
-
1a1. Cow shows an error message.
Use case ends.
-
Instructions for Manual Testing
Adding Tasks
-
Adding a task while all tasks are listed
-
Prerequisites: List all tasks using the
tasks list
command. There can be any number of tasks in the list. -
Test case:
tasks add n/Brush the cows sd/20180101 st/0000 ed/20181231 et/2359 t/Chill
Expected: A new task with the fields entered is added to the bottom of the task list. -
Test case:
tasks add n/Slaughter the cows ed/20190101 et/1200
Expected: A new task with the entered name and end date and time is added with the current date and time as the start date and time. -
Test case:
tasks add n/Feed the cows sd/20180101 st/2359 ed/20180101 et/0000
Expected: No task is added. An error message is shown. -
Test case:
tasks add n/Feed the cows ed/19700101 et/0000
Expected: No task is added. An error message is shown. -
Test case:
tasks add n/Feed the cows sd/20180101 st/0000 ed/20180231 et/2500
Expected: No task is added. An error message is shown. -
Other incorrect tasks add commands to try: Names containing non-alphanumeric characters, dates that don’t exist on the calendar, times outside the range 0000-2359 inclusive, wrongly formatted dates and times.
-